Breaking Out of the Iframe: Why We’re Building a Browser SDK for Full Flexibility
Deciding to build out a browser SDK changes our goal from delivering a best-in-class UX to also delivering an equally delightful developer experience.
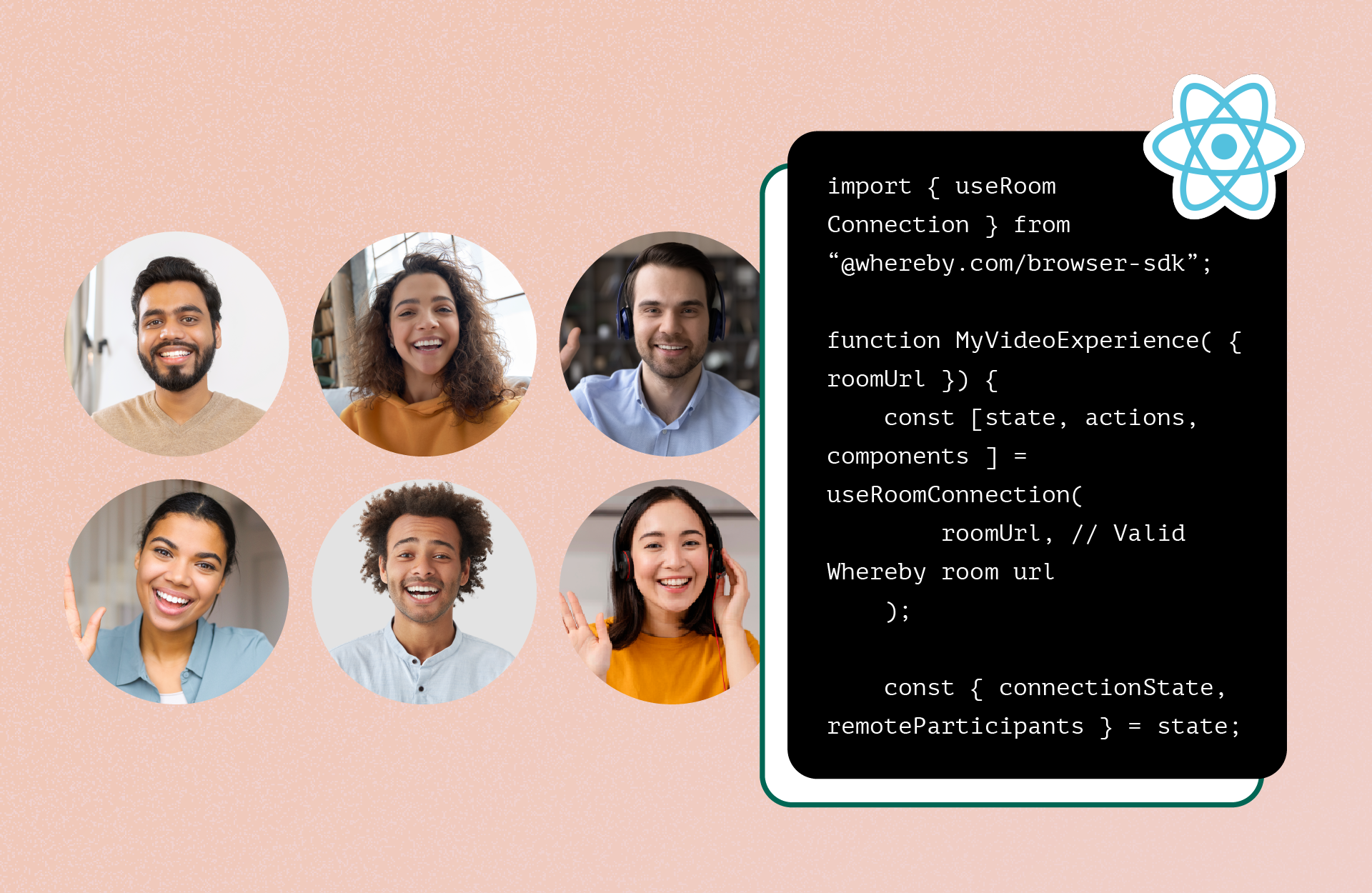
As engineers working for Whereby, we have always taken great pride in being able to work on and deliver one of the most frictionless video experiences on the market. As one of the first WebRTC services out there, we set the goal of making video conferencing as easy as picking up the phone. It is easy to forget, but not very long ago, this was not the case, and we believe we have played our part in making video conferencing as omnipresent as it is today.
We’re proud that our Whereby Meetings product is used by millions of users every month for hassle-free and enjoyable video calls directly in the browser.
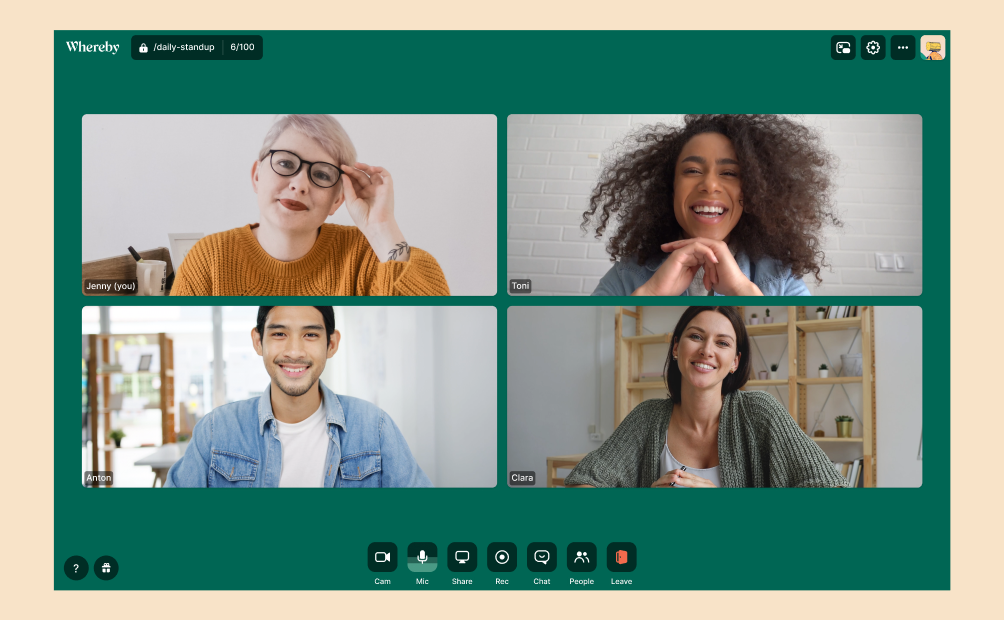
Over the years, our product and customer base have changed quite dramatically.
In addition to the general-purpose Whereby Meetings product we have always offered, we now focus fully on enabling other businesses to add video to their service through Whereby Embedded.
This real-time video conferencing API makes it super easy for developers to integrate Whereby’s much-loved UX into any website or app — we’ve seen great success stories in the telehealth space, for instance, but there are also compelling integrations in dating apps, recruiting products, e-learning platforms and more. And even though our easy-to-use UX is a pillar of our Embedded offering by enabling a blazing fast implementation, we have learnt that many of our customers want even more control over their own end-user video experience.

This puts us at a cross-road; do we continue delivering an increasingly flexible embedded solution, or do we take the plunge and deliver a full blown browser SDK for our customers to build exactly the experience they want?
We have landed on the latter, as we see this as the natural next addition to our offering. We will still develop a best-in-class embedded experience, but in addition unblock those of you who are building things we can’t even imagine.
Are you building out the next big online collaboration tool? Use our SDK to have the participants’ video tiles float around depending on what they are working on. The opportunities are infinite and provide builders with the flexibility needed to integrate real-time video conferencing in the best and most exciting ways.
Deciding to build out a browser SDK changes our goal from delivering the best-in-class UX to also delivering an equally delightful developer experience. Our belief is that an enjoyable SDK is one that seamlessly integrates with your existing framework and strikes a balance between exposing all the relevant functionality while hiding away the complexities you dont care about.
Knowing that many of our customers are using React, we have decided to start out with offering a React integration as part of our SDK. If you are building a React web app which needs video capabilities, our new offering should be the thing for you. Please read on for some of the more technical details.
How does it work?
In order to make our React integration work with most setups, we are going to expose a set of custom hooks which allows the developer to quickly hook into our service and set up the camera, microphone, and establish a connection to other participants in the same “room”. What is returned from the hooks is a state representation of the media / connection, alongside functions to change this state. Off the bat, we will include two such hooks, namely useLocalMedia
and useRoomConnection
.
The useLocalMedia
hook is added to enable our customers to build rich “pre-call” experiences, where end users are able to preview and change the devices they want to connect with. “No surprises” was our mantra when building out the Whereby pre-call experience, and we anticipate this rings true for most video use cases.
import { useLocalMedia } from "@whereby/browser-sdk/react";
function MyPreviewUX( { /* props */ }) {
const [state, actions, components ] = useLocalMedia({
audio: true, // Request mic access
video: true // Request cam access
});
const { localStream, devices, effects } = state;
const { toggleEffect, toggleMicrophone } = actions;
const { VideoView } = components;
return <div>
{ /* Render any UI, making use of state */ }
<VideoView stream={localStream} />
</div>;
}
Once local devices have been previewed and confirmed, the useRoomConnection
hook takes care of establishing a connection to the room, returning back the list of remote participants including their media streams. From this data you are free to render a grid, or non-grid if you like, of all the people on the call. Using the returned functions to toggle camera, microphone, and send chat messages, we hope you can build the experience which is best for your users.
import { useRoomConnection } from "@whereby/browser-sdk/react";
function MyVideoExperience( { roomUrl }) {
const [state, actions, components ] = useRoomConnection(
roomUrl, // Passed on from your servers
{ localStream }, // Passed on from eg useLocalMedia
);
const { connectionState, remoteParticipants } = state;
const { sendReaction } = actions;
const { VideoView } = components;
return <div className="videoGrid">
{ /* Render any UI */ }
{ remoteParticipants.map((p) => (
<VideoView key={p.id} stream={p.stream} />
)) }
</div>;
}
So where are we? Currently, we are iterating fast over a few alpha versions to get the core functionality added. Our latest alpha versions are available on npm, free for you to test out today. However, it is still very early days, and we would LOVE to hear from you and your requirements of such an SDK. Please submit an issue or join our Discord community to get in touch.
View our developer documentation