How We Used Our SDK To Build an Interactive Quiz Game in 2 Days
Find out how the Whereby team used a hackathon to test the limits of their SDK and created an interactive quiz game in just 2 days.
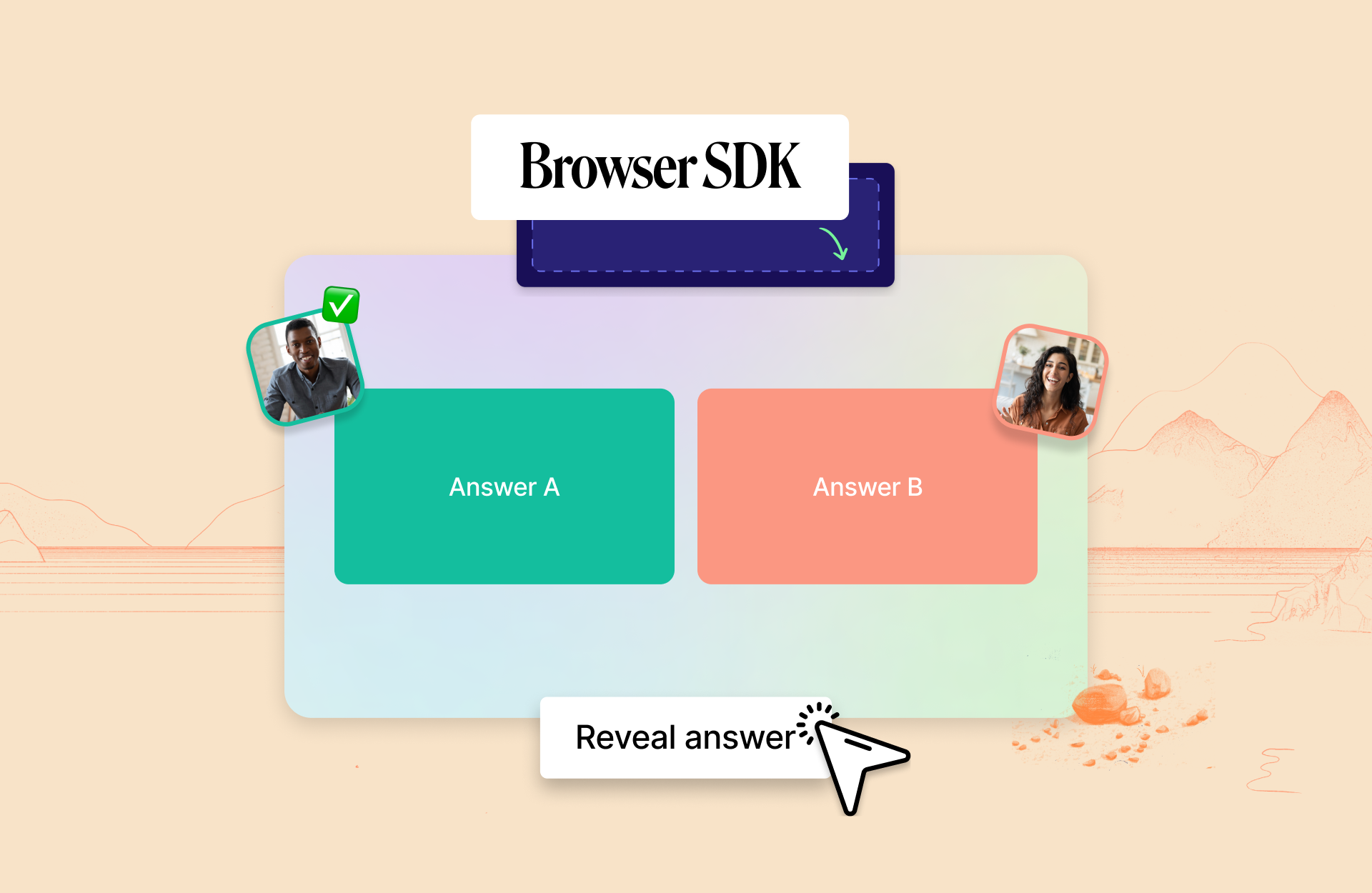
At Whereby, we have always taken pride in our ability to provide seamless video experiences to millions of people worldwide. However, we are constantly evolving to meet the changing needs of our customers.
Recently, we launched our Browser SDK, which enables our customers to create a customized experience with full control over the UI. While our pre-built UI solution provides a ready-to-use option that can be embedded in any website or app, the SDK allows you to break away from our pre-built video conferencing experience and create your own unique solution.
At a recent company hackathon, our team focused on an idea related to the SDK. We value innovation and creativity, and we also believe in using our own products at Whereby to identify areas for improvement. As a remote company, we particularly enjoy hackday events because they allow us to bring our ideas to life, collaborate more closely as a team, and have fun in the process.
So, what did we build?
Hackday Ideation
If you’re anything like me, during 2020 you spent a lot of time playing Jackbox-esque games online with friends.
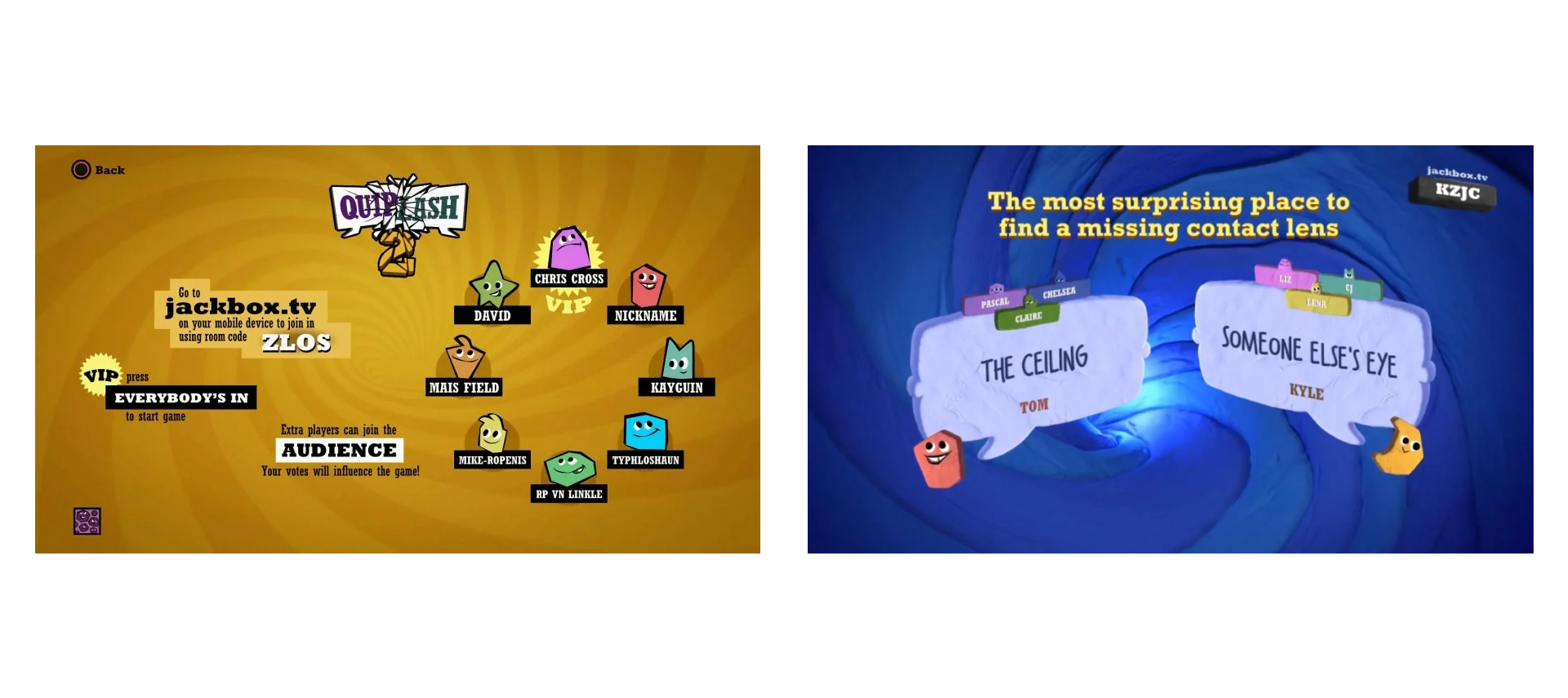
There are a few great things about Jackbox and similar games -
Loads of players can join the experience
The barrier to entry is low (just an internet device, no controller needed)
Interactions drive the real fun (chatting while playing)
The Browser SDK offers a significant level of control and flexibility, without the complications of dealing with WebRTC yourself. Simply take the video-call, break apart the visual components and make your own interface - from the video tiles, audio controls, chat interface and lots more.
What if we could build a similar question / answer based game, add audio and video communication to it - but instead of avatars, Whereby video tiles could fly around and interact with questions?
Hacking Away
Getting started with local video
We kicked off with a boilerplate React project, and used ChakraUI to prototype components quickly. First thing on the list was seeing your own camera in our app.
This was my first time using the browser-sdk package personally, and found it refreshingly easy to get started with. The useLocalMedia
hook is exposed, which allows us to render our own video stream, which you might use in a pre-game lobby, or device setup screen.
import { useLocalMedia } from "@whereby.com/browser-sdk";
import MyVideoTile from "components/MyVideoTile";
const DeviceSetup = () => {
const localMedia = useLocalMedia({ audio: true, video: true });
const { localStream, devices, effects } = localMedia.state;
const { toggleCameraEnabled, toggleMicrophoneEnabled } = localMedia.actions;
return (
<div>
{ /* Render UI for device setup & controls */ }
<MyVideoTile stream={localStream} />
</div>
)
Connecting with others
The other important building block of the SDK is the useRoomConnection
hook. Given a Whereby Embedded room URL, and your local video stream (available from useLocalMedia
), it sets up a connection, giving you access to the room state and other participants’ data, including their video streams.
import { useRoomConnection } from "@whereby.com/browser-sdk";
import Participants from 'components/participants';
// roomUrl is a Whereby Embedded room
const Game = ({ localMedia, displayName, roomUrl }: GameProps) => {
const roomConnection = useRoomConnection(roomUrl, {
localMedia,
displayName,
logger: console,
});
const { state: roomState } = roomConnection;
const { remoteParticipants, localParticipant, chatMessages } = roomState;
// Some game state logic
const { state: quizState, actions: quizActions } = useQuizGame(roomConnection);
return (
<div>
{quizState.screen === 'lobby' ? (
<LobbyView /> // Render appropriate screens
) : (
<GameView />
)
<ParticipantsGrid roomConnection={roomConnection} />
</div>
)
}
The pre-call screen using useLocalMedia
and the Lobby with other players. Similar to Jackbox, there is one person set as the game host, and players can join via a QR / link.
Game State
We needed to be able to communicate questions and answers between each player, and keep the game state in sync. Naturally, we thought to build out a dedicated WebSocket server to handle this.
However - In the interest of pushing the capabilities of the SDK, and simplifying deployment - we decided to leverage the chat functionality of the Whereby meeting as our messaging protocol.
Because under the hood, each player is actually a participant of a Whereby meeting, we had access to incoming messages through chatMessages
from roomState
.
We could parse these messages and pass them to our game state handler with relative ease - a surprising experiment that worked well for us!

This meant the game state was P2P, with no backend server storing questions / scores. This has a lot of limitations of course, and we'd recommend a dedicated WebSocket server for a production-ready app - but for a Hackathon we could move quickly this way.
Finishing touches
One of the most important distinctions in our idea was to be pretty wild with the user interface. We wanted it to actually feel like a game, rather than a typical video-call or web app. Building off of ChakraUI gave the project framer-motion
for free, which we used to create fun reactive animations.
Having the video tiles be dynamic was a crucial part of the experience. Given more time - we would've looked at other animations for eliminating players, team vs team and head to head rounds!
An MVP in two days
In the end, it wasn’t exactly a Jackbox game - but within a short hackathon, we had deployed a fully multiplayer game that you could join from any device, and play along with fully working audio and video. The hackday code is freely available on GitHub: https://github.com/whereby/hackday-sdk-demo
While this was just one project, there were a lot of amazing ideas during our hackathon, and it was incredible to see the flexibility of both Whereby Embedded, and the Browser SDK. We’d love for you to try out the Browser SDK too - and see what you can make with your imagination.
Our latest versions are available on npm, free to play with today with a Whereby Embedded account. We will continue to iterate and improve the core functionality and the developer experience, so we’d love more feedback and use cases as we grow this. Please submit an issue or join our Discord community to get in touch.
In the meantime, we’ll keep building a world in which anywhere works, but perhaps we’re also moving closer to a world in which anything works, too.